In C programming, dynamic memory allocation allows us to allocate memory at runtime. Two commonly used functions for this purpose are malloc
and calloc
. While they may seem similar, there are important differences between the two. This article explores these differences with examples.
Key Differences Between malloc
and calloc
Feature | malloc | calloc |
---|---|---|
Full Form | Memory Allocation | Contiguous Allocation |
Initialization | Does not initialize the allocated memory. Memory contains garbage values. | Initializes all allocated memory to zero. |
Parameters | Takes a single argument: the number of bytes to allocate. | Takes two arguments: the number of blocks and the size of each block. |
Performance | Slightly faster as it doesn’t initialize memory. | Slightly slower due to memory initialization. |
Syntax | void* malloc(size_t size) | void* calloc(size_t n, size_t size) |
Syntax and Usage
malloc
Example
Output:
Values in allocated memory:
“Garbage Values”
calloc
Example
Output:
Values in allocated memory:
0 0 0 0 0
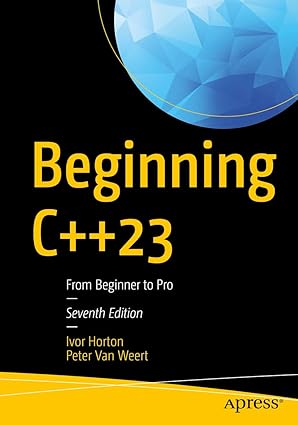
Kickstart your coding journey with Beginning C++23 – the ultimate guide to mastering the latest in modern C++ programming!
View on Amazon
Key Takeaways
- Use
malloc
when you don’t need the memory to be initialized and want faster allocation. - Use
calloc
when you need the allocated memory to be initialized to zero. - Both
malloc
andcalloc
require you to explicitly free the memory using thefree()
function to avoid memory leaks.
By understanding the differences between malloc
and calloc
, you can choose the right function for your specific use case. Proper use of these functions helps ensure efficient memory management in your programs.