This is a simple C# .NET Program to validate email address. The ValildateEmail() function checks for a valid email and returns true if the email address is a valid email otherwise it returns false if the email address is not proper syntax. The code is well commented and should explain what is happening .
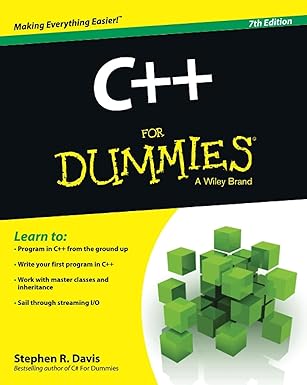
Unlock the world of programming with C++ for Dummies – the perfect beginner’s guide to mastering C++ with ease and confidence!
View on Amazon
This code uses regular expressions to validate the structure of the email address and checks for the presence of “@” and “.” characters. Additionally, it has a separate function ContainsIllegalCharacters
to check for the presence of any illegal characters in the email address.