As a programmer, mastering algorithms is essential for writing efficient, scalable, and maintainable code. Algorithms are the building blocks of problem solving in computer science and are used to manipulate, process, and analyze data. Whether you are developing software, optimizing systems, or solving complex computational problems, knowing the right algorithm to use can make all the difference.
In this article, we’ll explore the top 10 algorithms that every programmer should know, ranging from basic sorting techniques to sophisticated methods used in machine learning and artificial intelligence. Understanding these algorithms will not only sharpen your programming skills but also enhance your ability to tackle a wide variety of coding challenges.
- Sorting Algorithms
- Search Algorithms
- Dynamic Programming
- Divide and Conquer
- Greedy Algorithms
- Graph Algorithms
- Backtracking Algorithms
- Hashing Algorithms
- String Matching and Parsing Algorithms
- Machine Learning Algorithms
- FAQs
Sorting Algorithms
Sorting algorithms are essential for organizing data in a specific order, typically in ascending or descending. Quick Sort and Merge Sort are popular for their efficiency, while simpler algorithms like Bubble Sort provide a foundational understanding of sorting techniques.
Examples: Quick Sort, Merge Sort, Bubble Sort
Importance: Sorting is foundational in computer science and helps with organizing data efficiently.
Common Usage:
- E-commerce Platforms: Sorting algorithms like Quick Sort and Merge Sort are used in product listings on websites like Amazon, where items are sorted by price, ratings, or relevance.
- Database Management: Sorting is fundamental in database operations like indexing and querying large datasets. For example, SQL queries often use sorting algorithms to arrange records by certain attributes, such as dates or customer names.
Search Algorithms
Search algorithms are used to find specific elements within a data structure. Binary Search is efficient for searching in sorted arrays, while Depth-First Search (DFS) and Breadth-First Search (BFS) are key for exploring graph and tree structures.
Examples: Binary Search, Depth-First Search (DFS), Breadth-First Search (BFS)
Importance: These algorithms are crucial for finding elements in data structures like arrays, trees, and graphs.
Common Usage:
- Google Search: Binary Search is used behind the scenes for searching sorted indexes of web pages. It’s used for fast lookups of relevant results in response to a search query.
- Social Networks: DFS and BFS are commonly used in social network applications, such as Facebook or LinkedIn, to explore user connections, find friend recommendations, or traverse networks of connections.
Dynamic Programming
Dynamic programming is a method used to solve complex problems by breaking them down into simpler overlapping subproblems. It is ideal for optimization problems, like the Fibonacci sequence or the Knapsack problem where previously solved subproblems can be reused to avoid redundant calculations.
Examples: Fibonacci Sequence, Longest Common Subsequence, Knapsack Problem
Importance: Dynamic programming optimizes problems by breaking them into subproblems and solving them recursively.
Common Usage:
- Financial Services: The Knapsack problem, a common dynamic programming algorithm, is used in investment planning to maximize returns by selecting the best combination of assets given certain constraints (e.g., budget, risk).
- Game Development: Dynamic programming is used to optimize AI strategies in games. For example, in chess or checkers, algorithms like Minimax (enhanced with dynamic programming) help compute the best moves for the AI player.
Divide and Conquer
Divide and conquer algorithms work by breaking a large problem into smaller, more manageable subproblems. These smaller subproblems are solved independently, and then their solutions are combined to solve the original problem. For example, Merge Sort and Quick Sort algorithms split data into smaller parts, sort or solve each part separately, and finally merge the results efficiently.
Examples: Merge Sort, Quick Sort, Binary Search
Importance: This paradigm divides problems into smaller subproblems, solves them independently, and combines their solutions.
Common Usage:
- Data Processing: Merge Sort is widely used in systems that need to handle large amounts of data, such as database management systems (DBMS) or big data processing tools like Hadoop and Spark.
- Computational Geometry: Algorithms like Quick Sort and Merge Sort are used in geometry-related tasks, such as dividing a large set of points into smaller subsets for efficient processing in problems like finding the convex hull.
Greedy Algorithms
Greedy algorithms make optimal choices at each step with the hope of finding a global optimum. They are used in problems like Huffman coding for data compression or Dijkstra’s algorithm for finding the shortest path in a graph, where the local optimal choices lead to the global optimum.
Examples: Huffman Coding, Dijkstra’s Algorithm, Activity Selection Problem
Importance: These algorithms make optimal choices at each step to find global optima.
Common Usage:
- Network Routing: Dijkstra’s Algorithm is commonly used in networking, for example, in routing protocols (like OSPF and BGP) to find the shortest path between routers to send data packets efficiently.
- Huffman Coding: Used in data compression techniques, such as in ZIP files or MP3 files, Huffman coding helps minimize file sizes by encoding the most frequent characters with shorter binary codes.
Graph Algorithms
Graph algorithms are crucial for solving problems involving networks, such as social media connections, flight paths, or computer networks. Dijkstra’s algorithm finds the shortest path between nodes, while Kruskal’s and Prim’s algorithms are used for finding the minimum spanning tree in a graph.
Examples: Dijkstra’s Algorithm, Kruskal’s Algorithm, Bellman-Ford Algorithm
Importance: They solve network-based problems, such as shortest paths and minimum
Common Usage:
- Google Maps: Dijkstra’s Algorithm is used to calculate the shortest path between two locations, taking into account real-time traffic data for accurate travel time predictions.
- Telecommunications: Graph algorithms are used in network design, where companies like AT&T or Verizon use algorithms like Kruskal’s and Prim’s to design efficient telecommunications networks with minimum cost and optimal connectivity.
Backtracking Algorithms
Backtracking algorithms systematically explore all possible solutions to a problem and discard those that do not work. These are often used for constraint satisfaction problems. Problems like the N-Queens puzzle or Sudoku solvers can be efficiently tackled using backtracking.
Examples: N-Queens Problem, Sudoku Solver, Subset Sum Problem
Importance: Backtracking helps solve problems with constraints by exploring all possible solutions.
Common Usage:
- Puzzle Games: Solvers for puzzles like Sudoku or the N-Queens problem use backtracking to find the correct arrangement of numbers or queens on a board.
- Resource Allocation: Backtracking is used in scheduling problems or to assign resources to tasks where certain constraints must be satisfied (e.g., project scheduling with limited resources).
Hashing Algorithms
Hashing algorithms map data to fixed-size values, called hash codes, making data retrieval faster. Hash tables, hash maps, and cryptographic hash functions like SHA-256 are examples, providing efficient access and security for storing and retrieving data.
Examples: Hash Tables, Hash Maps, SHA-256
Importance: Hashing provides efficient data retrieval and is used in areas like databases and cryptography.
Common Usage:
- Data Storage: Hashing is widely used in databases and key-value stores like Redis or MongoDB, where data is mapped to specific hash values for efficient retrieval.
- Cryptography: Cryptographic algorithms like SHA-256 are used in securing communications (such as HTTPS) and in blockchain technologies, providing a secure way to ensure data integrity and immutability.
String Matching and Parsing Algorithms
String matching algorithms are used to search for patterns or substrings within larger strings. The Knuth-Morris-Pratt (KMP) and Rabin-Karp algorithms optimize the process by minimizing the number of comparisons, making them ideal for text processing tasks.
Examples: Knuth-Morris-Pratt (KMP) Algorithm, Rabin-Karp Algorithm
Importance: These algorithms are essential for searching patterns in text and parsing strings.
Common Usage:
- Text Search: The Knuth-Morris-Pratt (KMP) algorithm is used in text editors and word processors (e.g., Sublime Text, Microsoft Word) for efficient searching of substrings within large documents.
- Web Scraping: String matching algorithms are used in web scraping tools to extract relevant data from HTML documents, like extracting product prices from e-commerce websites or headlines from news articles.
Machine Learning Algorithms
Machine learning algorithms enable computers to learn from data and make predictions or decisions. Algorithms like K-Nearest Neighbors (KNN), Decision Trees, and Gradient Descent are the foundation for building predictive models in applications ranging from recommendation systems to image recognition.
Examples: K-Nearest Neighbors (KNN), Decision Trees, Gradient Descent
Importance: Machine learning algorithms enable systems to learn from data and improve over time.
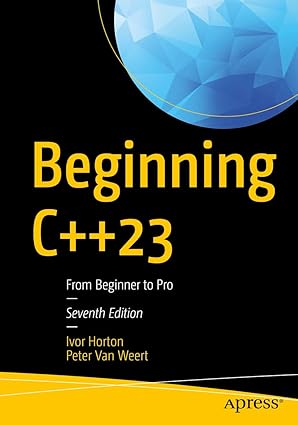
Kickstart your coding journey with Beginning C++23 – the ultimate guide to mastering the latest in modern C++ programming!
View on Amazon
Common Usage:
- Recommendation Systems: Algorithms like K-Nearest Neighbors (KNN) and Decision Trees are used by companies like Netflix or Amazon to recommend movies, TV shows, or products based on users’ past preferences and behaviors.
- Image Recognition: Machine learning algorithms, including Gradient Descent and Decision Trees, are extensively used in applications like facial recognition or object detection, powering systems in smartphones, security cameras, and autonomous vehicles.
FAQs
What is an algorithm?
An algorithm is a step-by-step procedure or formula used to perform a specific task or solve a problem. In computer science, algorithms are used to process data, perform calculations, and automate reasoning tasks efficiently.
Why are algorithms important in programming?
Algorithms are fundamental to programming because they provide efficient ways to solve problems. Knowing the right algorithm to use can drastically improve performance, reduce resource consumption, and make code more maintainable and scalable.
Which sorting algorithm is the fastest?
The speed of a sorting algorithm depends on the dataset and the context in which it is used. Quick Sort and Merge Sort are generally faster than others for large datasets, while algorithms like Bubble Sort or Insertion Sort are simpler but less efficient for large data.
When should I use a greedy algorithm?
A greedy algorithm is best used when the problem involves making a sequence of choices, where choosing the local optimum at each step leads to a globally optimal solution. It’s ideal for problems like activity selection or minimum spanning trees.
How do hashing algorithms improve performance?
Hashing algorithms allow for constant-time access to data by mapping it to a fixed-size hash code. This is particularly useful for implementing data structures like hash tables or hash maps, which enable fast insertion, deletion, and retrieval operations.
I use any algorithm in any problem?
Not all algorithms are suited for every problem. It’s crucial to analyze the problem characteristics, such as the type of data, the size of the input, and the required efficiency, before choosing the best algorithm for a specific task.