This C program shows insertion in Arrays. It shows how to insert an element in an array at any point.
This program also shows how to print the updated array after the item is inserted in the array.
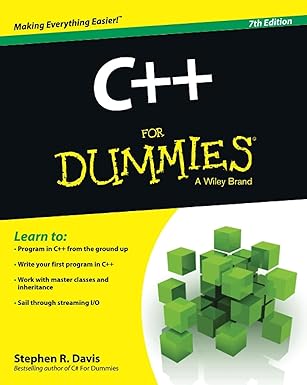
Unlock the world of programming with C++ for Dummies – the perfect beginner’s guide to mastering C++ with ease and confidence!
View on Amazon1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | #include<stdio.h> void main() { int a[10]; int i, n, j, item, m; printf("Enter an array of Ten Elements\n"); for (i = 1; i <= 10; i++) scanf("%d", & a[i]); printf("The array you enterd was\n"); for (i = 1; i <= 10; i++) printf("\n%d", a[i]); printf("\nEnter an element to insert in the array\n"); scanf("%d", & item); printf("\nEnter Location (1--10) to insert this element\n"); scanf("%d", & m); a[m] = item; printf("\nThe number was\n%d ", item); printf("\nLocation was\n%d ", m); printf("\nUpdated array is\n"); for (i = 1; i <= 10; i++) printf("%d\n ", a[i]); } |
The output of the Insertion in Arrays C program is:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | Enter an array of Ten Elements 1 3 4 5 6 7 8 9 0 3 5 The array you enterd was 1 3 5 6 7 8 9 0 3 5 Enter an element to insert in the array 99 Enter Location (1--10) to insert this element 3 The number was 99 Location was 3 Updated array is 1 3 99 6 7 8 9 0 3 5 |