I have been receiving lot of emails and requests through the forums and inline forums attached to the tutorials about how to print pyramids and diamonds in different formats.
So, here in this article I will demonstrate how you can print pyramids and diamonds using for loop and if condition using C Programming. Building a pyramid in c programming is quite easy, but you must have the understanding of how for loop works. With some slight modifications you can print different shapes as well.
Need more details about this code – Click here for an article on this topic.
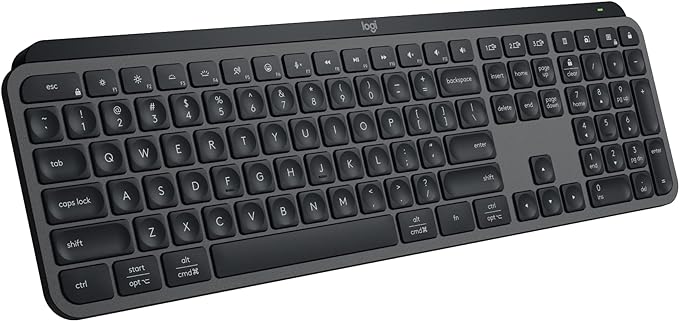
Experience precision and style with the Logitech MX Mechanical Wireless Keyboard – customizable backlighting, seamless connectivity, and ultimate typing comfort!
View on Amazon
This program is compiled and tested using Dev C++ compiler, however you can use any other C/C++ compiler with slight modifications in the code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 | #include <stdio.h> #include <conio.h> /*Program to build pyramids in pure c language With some slight modifications, different types of pyramids can be created. Numbers can also be used to build pyramids This program actually prints a diamond with * or numbers but with slight modifications, a pyramid can also b printed. */ int main(int argc, char * argv[]) { int i, k, n = 1, m; printf("\nPlease enter the number of lines for the pyramid\n"); printf("You can call it the radious of the pyramid"); printf("Number should be with in 1-30\n"); scanf("%d", & n); //This loop is used to print two print two pyramids //one of them will be updisde down to make a diamond. for (m = 0; m < 2; m++) { //loop through the number, the user has entered //loop to traverse through the pyramid for (i = 1; i <= n; i++) { //this block print spaces until the pyramid line starts if (m == 0) { //we are going to print the upper triangle for the diamond for (k = 1; k <= n - i; k++) { //it will print the spaces equal to the number entered by the user [1] printf(" "); } for (k = 1; k < 2 * i; k++) { //first line will print 1 * only and will increase by 1 each time [2] //its due to the value of i which is keep increasing 1 by 1 printf("%s", "*"); //printf("%d",i);//uncomment this line and comment above to print the numbers. } } //its the code to print the upside down triangles to complete the diamond. if (m == 1) { for (k = 1; k <= i; k++) { //its the reverse of the [1] to print 1 space in the beginning //and then increase the spaces as the loop counter increases. printf(" "); } for (k = 1; k < (n - i) * 2; k++) { //reverse code for [2], first it will print the * twice the the number which the user entered. printf("%s", "*"); //printf("%d",i); //uncomment this line and comment above to print the numbers. } } printf("\n"); } } system("PAUSE"); return 0; } |