This Java program creates a simple graphical application that draws rows of stars using Java’s Swing library. The program creates a window with rows of stars drawn on it. The Star
class defines the shape of a star, the StarPane
class handles the drawing logic, and the StarApplet
class sets up the main frame for the application. This example showcases basic GUI programming with Swing.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 | import javax.swing.*; import java.awt.*; import java.awt.geom.*; public class JavaStarsApplication extends JFrame { public JavaStarsApplication() { setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setTitle("Star App"); setSize(400, 400); Container content = getContentPane(); StarPane pane = new StarPane(); content.add(pane); setLocationRelativeTo(null); // Center the frame } // Class defining a pane on which to draw class StarPane extends JPanel { @Override protected void paintComponent(Graphics g) { super.paintComponent(g); Graphics2D g2D = (Graphics2D) g; Star star = new Star(0, 0); // Create a star float delta = 60f; // Increment between stars float starty = 0f; // Starting y position // Draw 3 rows of 4 stars for (int yCount = 0; yCount < 3; yCount++) { starty += delta; // Increment row position float startx = 0f; // Start x position in a row // Draw a row of 4 stars for (int xCount = 0; xCount < 4; xCount++) g2D.draw(star.atLocation(startx += delta, starty)); } } } public static void main(String[] args) { SwingUtilities.invokeLater(() -> { JavaStarsApplication applet = new JavaStarsApplication(); applet.setVisible(true); }); } } class Star { public Star(float x, float y) { start = new Point2D.Float(x, y); // store start point createStar(); } // Create the path from start void createStar() { Point2D.Float point = start; p = new GeneralPath(GeneralPath.WIND_NON_ZERO); p.moveTo(point.x, point.y); p.lineTo(point.x + 20.0f, point.y - 5.0f); // Line from start to A point = (Point2D.Float)p.getCurrentPoint(); p.lineTo(point.x + 5.0f, point.y - 20.0f); // Line from A to B point = (Point2D.Float)p.getCurrentPoint(); p.lineTo(point.x + 5.0f, point.y + 20.0f); // Line from B to C point = (Point2D.Float)p.getCurrentPoint(); p.lineTo(point.x + 20.0f, point.y + 5.0f); // Line from C to D point = (Point2D.Float)p.getCurrentPoint(); p.lineTo(point.x - 20.0f, point.y + 5.0f); // Line from D to E point = (Point2D.Float)p.getCurrentPoint(); p.lineTo(point.x - 5.0f, point.y + 20.0f); // Line from E to F point = (Point2D.Float)p.getCurrentPoint(); p.lineTo(point.x - 5.0f, point.y - 20.0f); // Line from F to g p.closePath(); // Line from G to start } Shape atLocation(float x, float y) { start.setLocation(x, y); // Store new start p.reset(); // Erase current path createStar(); // create new path return p; // Return the path } // Make the path available Shape getShape() { return p; } private Point2D.Float start; // Start point for star private GeneralPath p; // Star path } |
The output of the Java Program is:
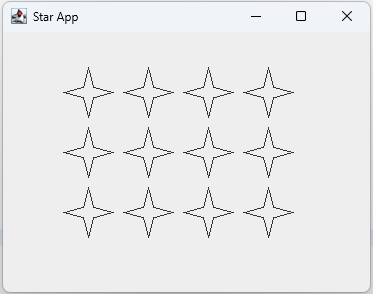
Java Program to draw Star Shapes