This source code shows you how to connect to Oracle Database 11g and execute queries using the PHP. First of all it sets the ORACLE_HOME and ORACLE_SID Environment variables using the putenv() method.
Note that to run this code, you will need PHP OCI8 extension enable in php.ini file.
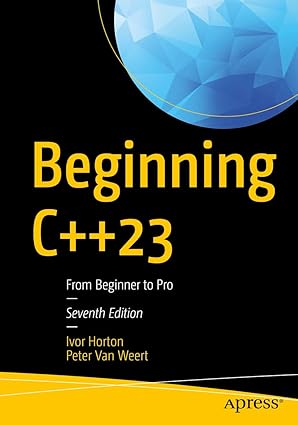
Kickstart your coding journey with Beginning C++23 – the ultimate guide to mastering the latest in modern C++ programming!
View on Amazon
Here are some of the common OCI8 functions which are used to perform different operations using the PHP Source Code.
Function | Description |
oci_bind_array_by_name | Binds PHP array to Oracle PL/SQL array by name |
oci_bind_by_name | Binds the PHP variable to the Oracle placeholder |
oci_cancel | Cancels reading from cursor |
oci_close | Closes Oracle connection |
oci_commit | Commits outstanding statements |
oci_connect | Establishes a connection to the Oracle server |
oci_define_by_name | Uses a PHP variable for the define-step during a SELECT |
oci_error | Returns the last error found |
oci_execute | Executes a statement |
oci_fetch_all | Fetches all rows of result data into an array |
oci_fetch_array | Returns the next row from the result data as an associative or numeric array, or both |
oci_fetch_assoc | Returns the next row from the result data as an associative array |
oci_fetch_object | Returns the next row from the result data as an object |
oci_fetch_row | Returns the next row from the result data as a numeric array |
oci_fetch | Fetches the next row into result-buffer |
oci_field_is_null | Checks if the field is NULL |
oci_field_name | Returns the name of a field from the statement |
oci_field_precision | Tell the precision of a field |
oci_field_scale | Tell the scale of the field |
oci_field_size | Returns the size of the field |
oci_field_type_raw | Tell the raw Oracle data type of the field |
oci_field_type | Returns data type of the field |
oci_free_statement | Frees all resources associated with statement or cursor |
oci_internal_debug | Enables or disables internal debug output |
oci_new_collection | Allocates new collection object |
oci_new_connect | Establishes a new connection to the Oracle server |
oci_new_cursor | Allocates and returns a new cursor (statement handle) |
oci_new_descriptor | Initializes a new empty LOB or FILE descriptor |
oci_num_fields | Returns the number of result columns in a statement |
oci_num_rows | Returns number of rows affected during statement execution |
oci_parse | Prepares Oracle statement for execution |
oci_password_change | Changes password of Oracle’s user |
oci_pconnect | Connect to an Oracle database using a persistent connection |
oci_result | Returns a field’s value from a fetched row |
oci_rollback | Rolls back outstanding transaction |
oci_server_version | Returns server version |
oci_set_prefetch | Sets number of rows to be prefetched |
oci_statement_type | Returns the type of an OCI statement |