This PHP script is designed to fetch and display detailed information about the fields within a MySQL database table. In simpler terms, it helps you visualize the structure of a table by printing a neat HTML table with essential details about each field, such as its name, data type, length, and various flags.
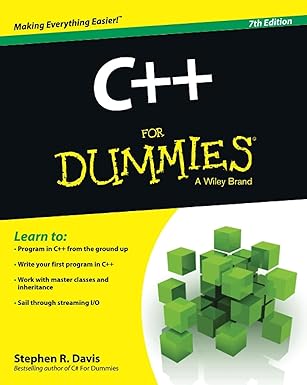
Unlock the world of programming with C++ for Dummies – the perfect beginner’s guide to mastering C++ with ease and confidence!
View on Amazon
The script uses MySQLi, a modern and more secure extension for interacting with MySQL databases in PHP. It provides a beginner-friendly way to understand the composition of your database tables. The script connects to the database, query the structure of a specific table and presents the information in an organized HTML format.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | <?php // Connect to the database $dbLink = mysqli_connect("localhost", "freetrade", "", "freetrade"); // Check the connection if (!$dbLink) { die("Connection failed: " . mysqli_connect_error()); } // Get list of fields $tableName = "invoice"; $query = "DESCRIBE $tableName"; $result = mysqli_query($dbLink, $query); // Start HTML table echo "<table>\n"; echo "<tr>\n"; echo "<th>Name</th>\n"; echo "<th>Type</th>\n"; echo "<th>Length</th>\n"; echo "<th>Flags</th>\n"; echo "</tr>\n"; // Loop over each field while ($row = mysqli_fetch_assoc($result)) { echo "<tr>\n"; echo "<td>" . $row['Field'] . "</td>\n"; echo "<td>" . $row['Type'] . "</td>\n"; echo "<td>" . $row['Length'] . "</td>\n"; echo "<td>" . $row['Null'] . " " . $row['Key'] . " " . $row['Default'] . " " . $row['Extra'] . "</td>\n"; echo "</tr>\n"; } // Close HTML table echo "</table>\n"; // Close the connection mysqli_close($dbLink); ?> |