Declaring a Class
After defining a class, you can declare it as a variable using the same syntax we have used for any other variable. A class is declared using its name followed by a name for the defined variable and ending with a semi-colon. For example, our ShoeBox class can be declared as follows:
When an object has been declared, you can access any of its members using the member access operator “.”. First, type the name of the object variable, followed by a period, followed by the name of the member you want to access. For example, to access the member Length of the above class, you would write:
Shake.Length;
Using this syntax, you can display the value of a class member:
cout << Shake.Length;
or you can request its value from the user, using the cin operator. Here is an example:
cin >> Shake.Lengh;
Using the
cout
extractor to display the values of the object members, our program could be as follows:
At this time, because of trying to access a private member, the program would produce the following error
[C++ Error] Unit1.cpp(30): E2247 ‘ShoeBox::TShoeSize’ is not accessible
Even if you change the ShoeSize member access from private to public, the program would render unpredictable results because the members have not been given appropriate values:
Characteristics of this shoe box Length = 0 Width = 1.79571e-307 Height = 4.17266e-315 Volume = 0 Color = Size = 3.58732e-43
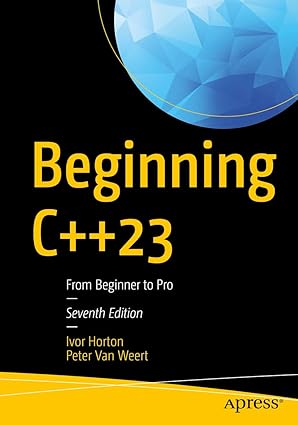
Kickstart your coding journey with Beginning C++23 – the ultimate guide to mastering the latest in modern C++ programming!
View on Amazon
Press any key to continue…