Pointers are a foundational concept in C and C++ that allows developers to manage memory and manipulate objects dynamically. However, traditional pointers come with challenges such as memory leaks, dangling pointers, and complex manual memory management. To address these issues, modern C++ introduces smart pointers, a powerful abstraction that automates memory management and ensures resource safety.
If you are new to pointers, you may want to revisit the foundational concepts of Pointers in C before diving into smart pointers.
- What Are Smart Pointers?
- Types of Smart Pointers in C++
- Advantages of Smart Pointers
- Difference Between Pointers and Smart Pointers
- Best Practices with Smart Pointers
- Use Cases for Smart Pointers
- Conclusion
What Are Smart Pointers?
Smart pointers are objects in C++ that encapsulate a raw pointer and automatically manage its lifetime. They use RAII (Resource Acquisition Is Initialization) principles to ensure that resources are properly released when they are no longer needed.
Unlike traditional pointers, smart pointers take care of memory deallocation, reducing the risk of memory-related issues like leaks or dangling references. They are part of the C++ Standard Library and provide a safer alternative to raw pointers.
Types of Smart Pointers in C++
The C++ Standard Library offers three primary types of smart pointers. The auto_ptr has been depreciated as of C++11.
1. std::unique_ptr
- Provides exclusive ownership of a dynamically allocated object.
- Ensures that only one
std::unique_ptr
instance manages a resource. - Ownership can be transferred using
std::move
, but copying is not allowed.
The unique_ptr Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 | #include <iostream> #include <memory> int main() { std::unique_ptr<int> ptr = std::make_unique<int>(42); std::cout << "Value: " << *ptr << std::endl; // Transfer ownership std::unique_ptr<int> newPtr = std::move(ptr); if (!ptr) std::cout << "Original pointer is null." << std::endl; return 0; } |
2. std::shared_ptr
- Allows shared ownership of a resource through reference counting.
- The resource is deallocated only when the last
std::shared_ptr
owning it is destroyed.
The shared_ptr Example:
1 2 3 4 5 6 7 8 9 10 11 12 | #include <iostream> #include <memory> int main() { std::shared_ptr<int> ptr1 = std::make_shared<int>(42); std::shared_ptr<int> ptr2 = ptr1; // Shared ownership std::cout << "Value: " << *ptr1 << std::endl; std::cout << "Use count: " << ptr1.use_count() << std::endl; return 0; } |
3. std::weak_ptr
- Provides a non-owning reference to a
std::shared_ptr
. - Useful for breaking cyclic dependencies in shared resources.
The weak_ptr Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 | #include <iostream> #include <memory> int main() { std::shared_ptr<int> shared = std::make_shared<int>(42); std::weak_ptr<int> weak = shared; // Non-owning reference if (auto ptr = weak.lock()) { // Check if resource is still available std::cout << "Value: " << *ptr << std::endl; } return 0; } |
Advantages of Smart Pointers
- Automatic memory management: Smart pointers automatically deallocate memory to prevent memory leaks.
- Safety: Prevent dangling pointers by ensuring proper resource cleanup.
- Improved readability: Simplify code by removing the need for explicit
delete
calls. - Shared ownership: Facilitate safe sharing of resources using
std::shared_ptr
. - Wild Pointers: Wild pointers are pointers that are declared and allocated memory but the pointer is never initialized to point to any valid object or address.
Difference Between Pointers and Smart Pointers
Aspect | Pointers | Smart Pointers |
---|---|---|
Memory Management | Manual using new and delete | Automatic using RAII principles |
Ownership | Can be shared or unmanaged | Clearly defined ownership types |
Safety | Prone to memory leaks, dangling pointers | Prevents memory leaks and dangling pointers |
Standard Library Support | Not part of the standard library | Part of the C++ Standard Library |
Complexity | Requires explicit cleanup | Simplifies memory management |
Reference Counting | Not available | Available in std::shared_ptr |
Best Practices with Smart Pointers
- Prefer
std::make_unique
andstd::make_shared
for creating smart pointers. These functions are safer and more efficient. - Avoid mixing raw pointers and smart pointers to prevent undefined behavior.
- Use
std::weak_ptr
judiciously to handle cyclic dependencies without unintended resource retention. - Overhead:
std::shared_ptr
incurs a slight performance cost due to reference counting. Use only when shared ownership is required. - Improper Usage: Misusing
std::weak_ptr
can lead to dangling references if not locked properly. - Overusing Smart Pointers: Not all pointers need to be smart; sometimes raw pointers or references suffice.
Use Cases for Smart Pointers
Resource Management:
- Managing file handles, sockets, and dynamic memory.
- Example: Using
std::unique_ptr
to manage file pointers or database connections.
Safe Ownership Transfer:
- Passing ownership safely between functions or objects.
- Example: Factory functions returning
std::unique_ptr
.
Breaking Cyclic Dependencies:
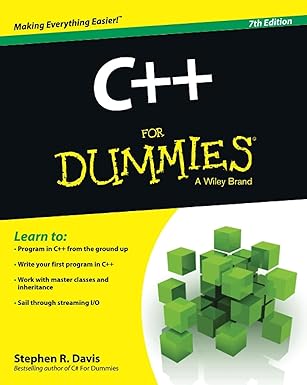
Unlock the world of programming with C++ for Dummies – the perfect beginner’s guide to mastering C++ with ease and confidence!
View on Amazon
- Using
std::weak_ptr
in graph-like structures to prevent reference cycles.
Conclusion
Smart pointers are a vital tool in modern C++ for managing dynamic memory safely and efficiently. They eliminate many pitfalls associated with raw pointers to make your C++ code more robust and easier to maintain. By understanding and applying smart pointers appropriately you can write cleaner, safer, and more efficient C++ programs.