Data structures are the foundation of efficient programming. They help store, organize, and manage data effectively. Choosing the right data structure improves code performance and optimizes resource usage. C++ offers a wide range of data structures that help solve different computational problems.
Significance of Data Structures
Efficient programming depends on selecting the right data structure for a given task. Data structures affect the speed and memory usage of a program. Proper use of data structures leads to faster execution and better resource management. Without the right data structures, even simple tasks become inefficient.
Importance of Efficient Data Handling in Programming
Efficient data handling ensures smooth program execution and optimal resource utilization. Poor data handling leads to slow performance, memory leaks, and unnecessary computational overhead. Efficient data structures help in managing large datasets, reducing processing time, and improving overall system performance. By implementing structured data management techniques, developers can enhance software efficiency and scalability.
Types of Data Structures in C++
C++ provides several built-in and user-defined data structures. The most commonly used ones include:
1. Arrays
Arrays store elements of the same type in a contiguous memory block. They allow fast access using an index. However, their size is fixed at the time of declaration.
Key Points:
- Fixed size.
- Fast access via index.
- Inefficient for insertions and deletions.
Why Use Arrays?
Use arrays in C++ for fast, indexed access to a fixed-size collection of elements with minimal memory overhead.
Example:
2. Linked Lists
Linked lists consist of nodes connected by pointers. Each node has data and a pointer to the next node. Unlike arrays, linked lists allow dynamic memory allocation.
Key Points:
- Dynamic size.
- Efficient insertions and deletions.
- Extra memory required for pointers.
Why use Linked Lists?
Use linked lists when you need dynamic memory allocation and efficient insertions or deletions without shifting elements.
Example:
3. Stacks
Stacks follow the Last In, First Out (LIFO) principle. The push()
operation adds an element, and the pop()
operation removes the top element.
Key Points:
- LIFO order.
- Used in function calls and undo mechanisms.
- Limited access (only top element).
Why use Stacks?
Use stacks for last-in, first-out (LIFO) operations, ideal for recursion, expression evaluation, and function call management.
Example:
4. Queues
Queues follow the First In, First Out (FIFO) principle. Elements are inserted at the back and removed from the front.
Key Points:
- FIFO order.
- Used in scheduling and buffering.
- Limited access (only front and back).
Why use Queues?
Use queues for first-in, first-out (FIFO) processing, making them essential for task scheduling and buffering.
Example:
5. Hash Tables (Unordered Maps)
Hash tables store key-value pairs for fast access. They use hash functions to map keys to indices.
Key Points:
- Fast lookups.
- Key-value storage.
- Hash collisions may occur.
Why use Hash Tables?
Use hash tables for fast key-value lookups, ensuring efficient data retrieval in constant average time.
Example:
6. Trees
Trees represent hierarchical structures. The most common type is the binary tree, where each node has up to two children.
Key Points:
- Hierarchical data structure.
- Fast searching, insertion, and deletion.
- Used in databases and file systems.
Why use Trees?
Use trees for hierarchical data representation, efficient searching, and optimized insertion and deletion operations.
Example:
7. Graphs
Graphs represent relationships between entities using nodes and edges. They are useful for modeling networks, maps, and social connections.
Key Points:
- Nodes and edges representation.
- Used in networking and route optimization.
- Can be directed or undirected.
Why use Graphs?
Use graphs to model complex relationships, such as networks, dependencies, and shortest path calculations.
Example:
Choosing the Right Data Structure
Choosing the correct data structure depends on the problem requirements. Consider these factors:
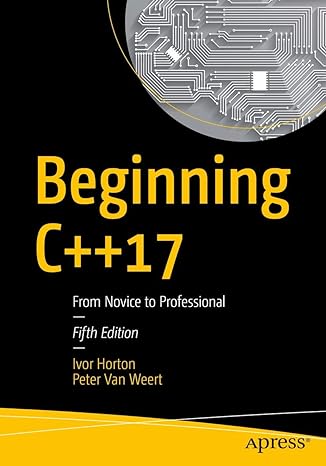
Master modern programming with Beginning C++17 – your gateway to building powerful, efficient, and future-ready applications!
View on Amazon
- Access Time: Arrays provide fast access, while linked lists require traversal.
- Insertion/Deletion: Linked lists are better for frequent insertions, while arrays are costly for resizing.
- Memory Usage: Dynamic data structures like linked lists use more memory due to pointers.
- Search Speed: Hash tables provide fast lookups, while linear search is slower.
Conclusion
Data structures play a vital role in efficient programming. Using the right data structure improves speed and resource management. C++ provides many built-in and user-defined data structures suitable for various tasks. Understanding their strengths and limitations helps in writing optimized and efficient programs.